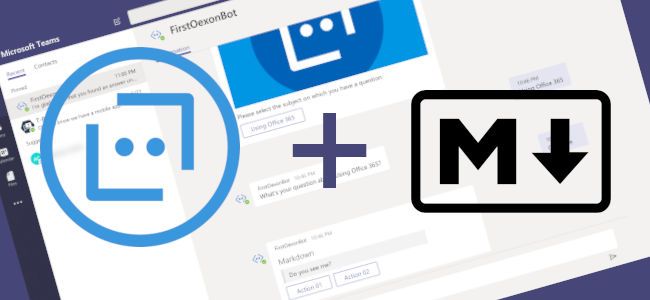
Display Markdown to your Bot Framework Hero and Thumbnail Cards π‘
I recently had the opportunity to really develop a Bot based on Microsoft Bot Framework that could work on Microsoft Teams. Without speaking about some aspects and technical choices, the principle of this Bot is simply to try to answer the questions of users asked on detailed thematics (Nothing too complex, you may say).
[note]Note
I choose to use the NodeJS solution (because I'm working on MacOS π).
All answers come from various sources and are mostly formatting thanks to Markdown. Everything is fine when the content is directly sent a message:
await turnContext.sendActivity(`# Markdown \n\n > Do you see me?`);
The example of code above, send a message to the user formatted in Markdown.
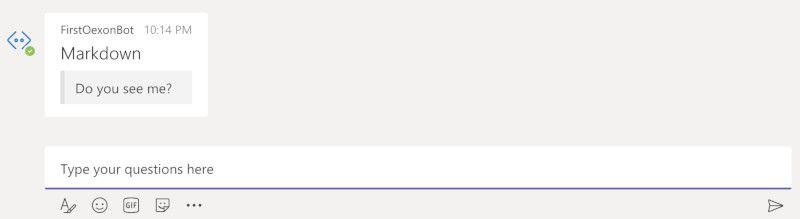
The following idea is to format the answers thanks to CardFactory (add some actions, title, or whatever...) π‘π±
displayAnswer(answer) {
return CardFactory.thumbnailCard(
'', // Title
[{}], // Image
[{
type: ActionTypes.ImBack,
title: 'Action 01',
value: 'One'
},
{
type: ActionTypes.ImBack,
title: 'Action 02',
value: 'Two'
}],
{
text: `# Markdown \n\n > Do you see me?`
});
}
The problem is the text is not formatted anymore and the Markdown tags are visible π€―
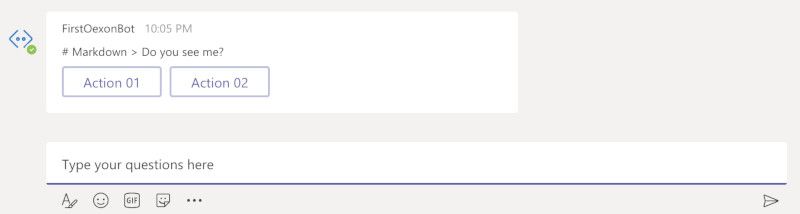
After some hours of research, I found an issue in GitHub in this regard:
The conclusion is (currently): HeroCards and ThumbnailsCard do not support the Markdown π€¨.
During my research, I have realized that HTML-rich text works! Great news, I can call all of my teammates and ask them to rewrite all documentation in HTML... Bad story. Finally, I also said to myself that may be possible to convert the Markdown content to HTML?
Eureka! I found a Framework named showdown that is "a Javascript Markdown to HTML converter, based on the original works by John Gruber" (John Gruber in addition! π―).
This Framework is awesome and it saved my day... I will explain to you how I used it.
First, install the package to your solution:
npm i showdown --save
Next, add the reference and create an object instance of showdown to your target javascript file:
const showdown = require('showdown');
[...]
let converter = new showdown.Converter();
Convert your Markdown text to HTML:
converter.makeHtml('# Markdown \n\n > Do you see me?')
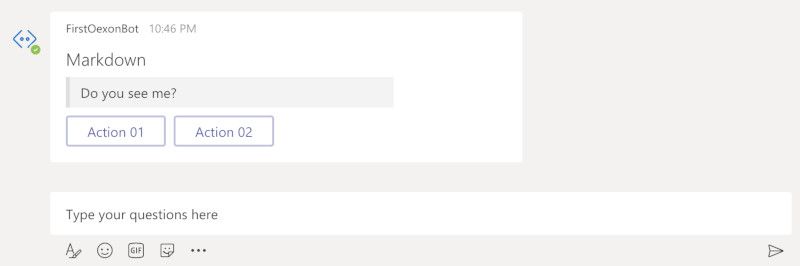
The full code of my function is the following one:
displayAnswer(answer) {
let converter = new showdown.Converter();
return CardFactory.thumbnailCard(
'', // Title
[{}], // Image
[{
type: ActionTypes.ImBack,
title: 'Action 01',
value: 'One'
},
{
type: ActionTypes.ImBack,
title: 'Action 02',
value: 'Two'
}],
{
text: `${converter.makeHtml('# Markdown \n\n > Do you see me?')}`
});
}
Hoping this post will help you π