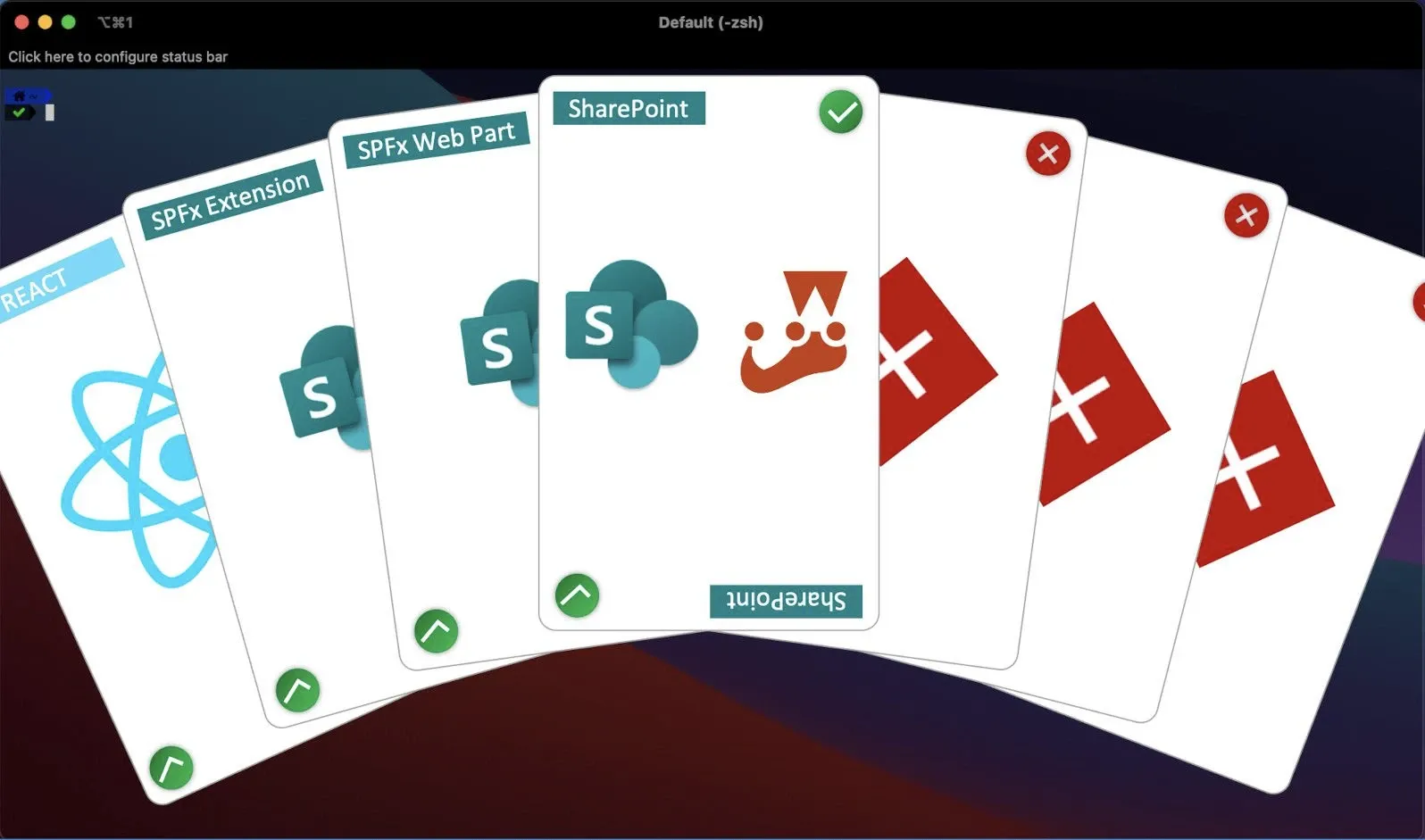
SharePoint Framework Unit Tests - React
In my previous post that talks about SharePoint Framework Unit Tests - TypeScript, the Enzyme tests were not a part of it.
Jest allows you to perform the test against the TypeScript files only but not to cover the React part (.tsx
files). To perform the React tests it is necessary to add another framework in addition to Jest - Enzyme.
How do these kinds of tests work? Thanks to Enzyme, you can isolate, manipulate and simulate output tests against your React Components'. In other words, Enzyme generates a "fake" DOM to inject your component and manipulate it.
[info]Note
This post is the continuity of SharePoint Framework Unit Tests - TypeScript
Prerequisites
- SharePoint Framework: 1.11.0 & 1.14.0
- NodeJS: 10.x & 14.x
Setup
The minimal setup to use Enzyme to perform the tests against your React files is:
- Sample-based on the existing Web Part created on the post SharePoint Framework Unit Tests - TypeScript
- For this example, you can keep the default React file located into
src/webparts/yourWebpart/component/
- Rather than creating a folder named test, I suggest you create a test file in the same location as the React (
.tsx
) one; create a file**.test.tsx
with this content:
/// <reference types="jest" />
import * as React from 'react';
import { configure, mount, ReactWrapper } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
configure({ adapter: new Adapter() });
import { IHelloTestsProps } from './IHelloTestsProps';
import HelloTests from './HelloTests';
describe('Hello Tests', () => {
let reactComponent: ReactWrapper<IHelloTestsProps, {}>;
afterEach(() => {
reactComponent.unmount();
});
it('should root web part element exists', () => {
reactComponent = mount(React.createElement(
HelloTests,
{
description: 'Description property value'
}
));
let cssSelector: string = '.helloTests';
const element = reactComponent.find(cssSelector);
expect(element.length).toBeGreaterThan(0);
});
});
NPM Packages
Before configuring and executing the unit tests, it is necessary to install the Enzyme Framework packages, that allow running the React unit tests:
- SPFx 1.11.0 - The Framework packages that allow running the unit tests:
npm i [email protected] [email protected] @types/[email protected] @types/[email protected] --save-dev
- SPFx 1.14.0 - The Framework packages that allow running the unit tests:
npm i enzyme enzyme-adapter-react-16 @types/enzyme @types/enzyme-adapter-react-16 jsdom-global jsdom --save-dev
[info]Important
- The versions of the packages are very important with a SPFx solution (v1.11.0)
- For the latest versions of SPFx, additional packages are required
- If you reuse the same configuration as the previous post (SharePoint Framework Unit Tests - TypeScript), you can launch the tests with the bash command below:
npm run test:jest
- If you launch the tests, this error should occur:
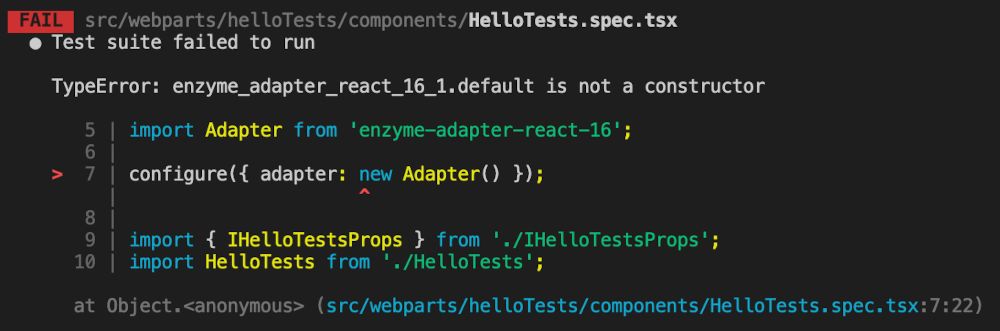